Hi guys, in this article we will discuss how to monitor the DHT sensor values in the Blynk application. For this we should have an account in the Blynk app, we can signup into Blynk either by Gmail or Facebook. After signup create a project with text labels, and we get an auth key to be placed in the code to post the values.
Let’s get started, in this article python script is used to read the DHT11 sensor values and post these values to the Blynk Cloud.
Components Required:
- Raspberry Pi
- SD card (Raspbian OS Installed)
- DHT11
Schematic:
Connections for DHT11 to Raspberry Pi
VCC – 5V
OUT – 23
GND – GND
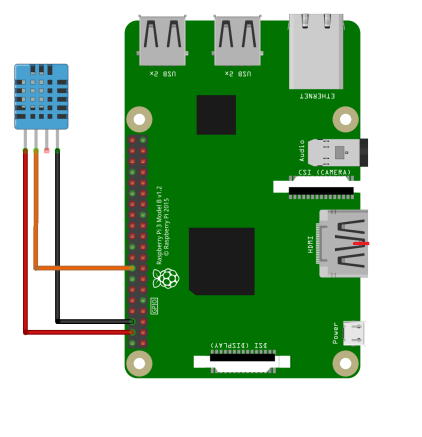
Code:
import blynklib
import Adafruit_DHT as dht
dht_pin = 23
BLYNK_AUTH = '<YOUR TOKEN>' #keep your token here
blynk = blynklib.Blynk(BLYNK_AUTH)
THRESH = 20
T_COLOR = '#f5b041'
H_COLOR = '#85c1e9'
ERR_COLOR = '#444444'
THRESH_COLOR = '#c0392b'
MSG = 'Low TEMP!!!'
T_PIN = 22
H_PIN = 21
class Counter:
cycle = 0
@blynk.handle_event('read V22')
def read_virtual_pin_handler(pin):
humidity, temperature = Adafruit_DHT.read_retry(dht.DHT11, dht_pin, retries=5, delay_seconds=1)
Counter.cycle += 1
if all([humidity, temperature]):
print('temperature={} humidity={}'.format(temperature, humidity))
if temperature <= THRESH:
blynk.set_property(T_PIN, 'color', THRESH_COLOR)
if Counter.cycle % 6 == 0:
blynk.notify(MSG)
Counter.cycle = 0
else:
blynk.set_property(T_PIN, 'color', T_COLOR)
blynk.set_property(H_PIN, 'color', H_COLOR)
blynk.virtual_write(T_PIN, temperature)
blynk.virtual_write(H_PIN, humidity)
else:
print('[ERROR] reading DHT11 sensor data')
blynk.set_property(T_VPIN, 'color', ERR_COLOR)
blynk.set_property(H_VPIN, 'color', ERR_COLOR)
while True:
blynk.run()
Output Screenshot:

